There´s pretty much nothing hard to explain. I´ve just simply created a structure from wood and iron hinges. Most important part of it is Arduino Leonardo microcomputer. I can easily press switch and Arduino Leonardo will send keyboard shortcut to my computer. Then I can for example mute/unmute microphone in Microsoft Teams or wherever my online class is.
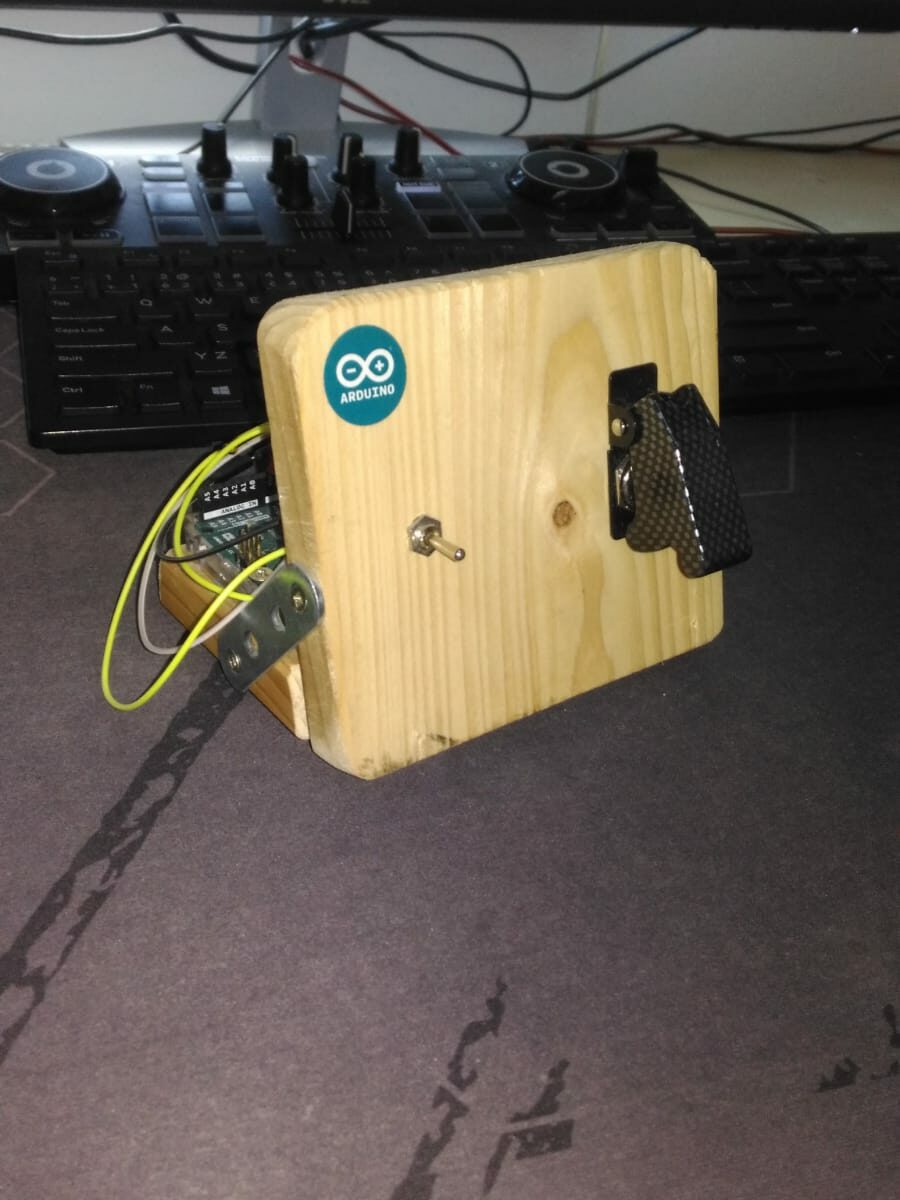
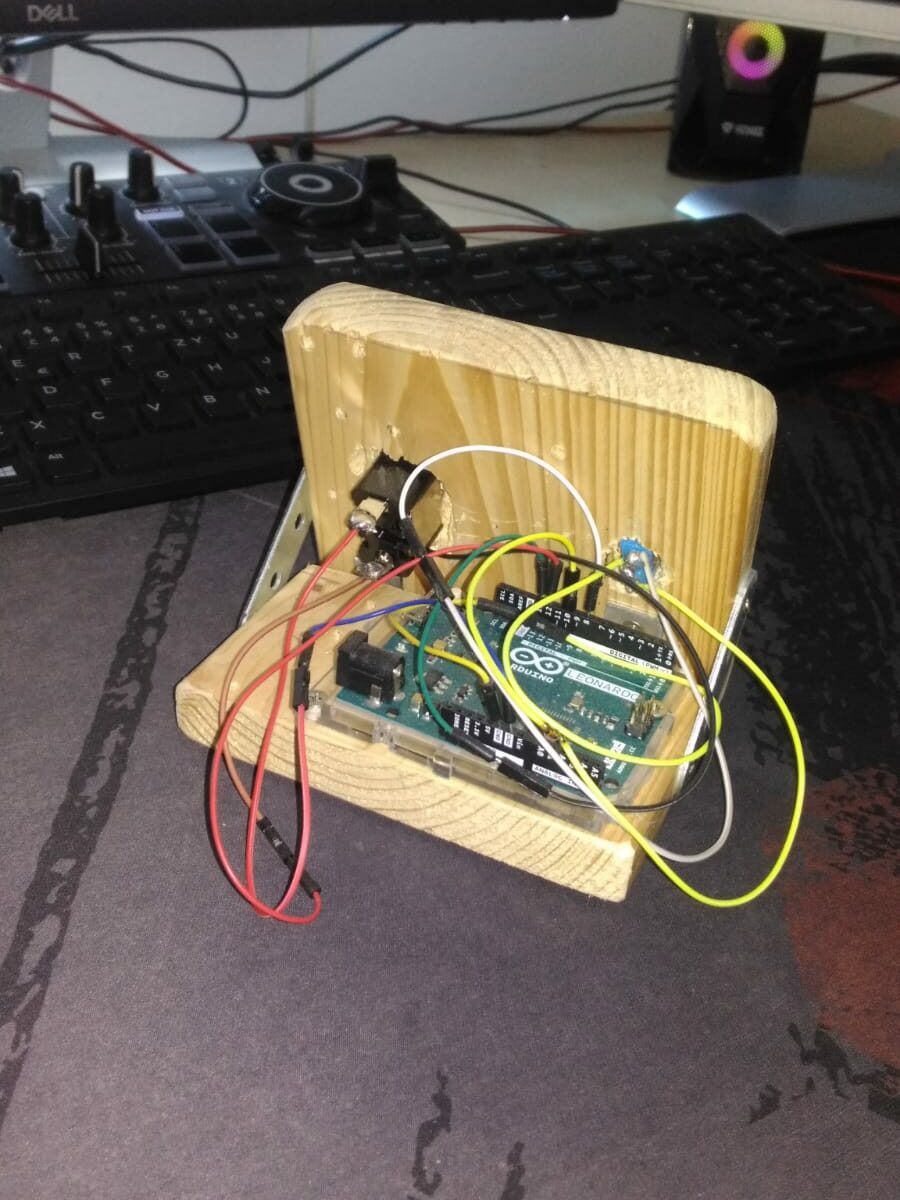
Code:
#include <Keyboard.h>
char ctrlKey = KEY_LEFT_CTRL;
char shftKey = KEY_LEFT_SHIFT;
char winKey = KEY_LEFT_GUI;
const int BUTTON_PIN = 8;
const int BUTTON_PIN2 = 10;
const int BUTTON_PIN3 = 12;
const int DEBOUNCE_DELAY = 50;
const int DEBOUNCE_DELAY2 = 50;
const int DEBOUNCE_DELAY3 = 50;
int lastSteadyState = LOW;
int lastFlickerableState = LOW;
int currentState;
int lastSteadyState2 = LOW;
int lastFlickerableState2 = LOW;
int currentState2;
int lastSteadyState3 = LOW;
int lastFlickerableState3 = LOW;
int currentState3;
unsigned long lastDebounceTime = 0;
unsigned long lastDebounceTime2 = 0;
unsigned long lastDebounceTime3 = 0;
void setup() {
pinMode(BUTTON_PIN, INPUT_PULLUP);
pinMode(BUTTON_PIN2, INPUT_PULLUP);
pinMode(BUTTON_PIN3, INPUT_PULLUP);
Keyboard.begin();
}
void loop() {
currentState = digitalRead(BUTTON_PIN);
currentState2 = digitalRead(BUTTON_PIN2);
currentState3 = digitalRead(BUTTON_PIN3);
if (currentState != lastFlickerableState)
lastDebounceTime = millis();
lastFlickerableState = currentState;
}
if (currentState2 != lastFlickerableState2)
lastDebounceTime2 = millis()
lastFlickerableState2 = currentState2;
}
if (currentState3 != lastFlickerableState3) {
lastDebounceTime3 = millis();
lastFlickerableState3 = currentState3;
}
if ((millis() - lastDebounceTime) > DEBOUNCE_DELAY) {
if(lastSteadyState == HIGH && currentState == LOW) {
delay(100);
Keyboard.releaseAll();
// Keyboard.println("You pressed the button ");
Keyboard.press(ctrlKey);
Keyboard.press(shftKey);
Keyboard.press('m');
delay(100);
Keyboard.releaseAll();
}
lastSteadyState = currentState;
}
if ((millis() - lastDebounceTime2) > DEBOUNCE_DELAY2) {
if(lastSteadyState2 == HIGH && currentState2 == LOW) {
Keyboard.releaseAll();
delay(100);
// Keyboard.println("You pressed the button ");
Keyboard.press(ctrlKey);
Keyboard.press(shftKey);
Keyboard.press('m');
delay(100);
Keyboard.releaseAll();
if ((millis() - lastDebounceTime3) > DEBOUNCE_DELAY3) {
if(lastSteadyState3 == HIGH && currentState3 == LOW) {
Keyboard.releaseAll();
delay(100);
// Keyboard.println("You pressed the button ");
Keyboard.press(ctrlKey);
Keyboard.press(shftKey);
Keyboard.press('o');
delay(100);
Keyboard.releaseAll();
}
lastSteadyState = currentState;
lastSteadyState2 = currentState2;
lastSteadyState3 = currentState3;
}
}
}